Delegates are everywhere in modern code, a delegate is a type that represents references to methods with a particular parameter list and return type. Delegates are used to pass methods as arguments to other methods. For example, event handlers are essentially methods that are invoked through delegates. Delegates actually remind me of C++ function pointers, but of course delegates are fully object-oriented.
There are few ways to represent delegates there is, for example, Func delegate. This generic delegate represents a method that takes one or more parameters and returns a value of a specified type. Here is an example (with a lambda expression):
Func<int, int> Multiplier = n => n * 5; int val = Multiplier(5); Console.WriteLine(val);
I believe the most recent variation of the delegate concept is Action
Action<string> outputFunc = GetOutputRoutine(); outputFunc("Hello, World!"); static Action<string> GetOutputRoutine() { return MyConsoleWriter; } static void MyConsoleWriter(string input) { Console.WriteLine("Console: {0}", input); }
So, this is a nice lesson, but why am I bringing all this up? While I find passing methods around like parameters is awfully convenient when writing code I also wish it was easier to follow while debugging. You can easily step into these methods but I often want to quickly navigate to the underlying code represented by the delegate without stepping, and with the latest updates to Visual Studio (17.10) it’s actually really easy.
Now when you are paused at the debugger you can hover over any delegate and get a convenient Go to source link, here is an example with Func.
Of course the source link in the above example goes to the line above, but you hopefully get my point.
Here is another example this time with the Action
The text makes it clear that going to source would ultimately redirect you to the MyConsoleWriter() method, even though we are directly pointing to GetOutputRoutine().
Love this new feature!
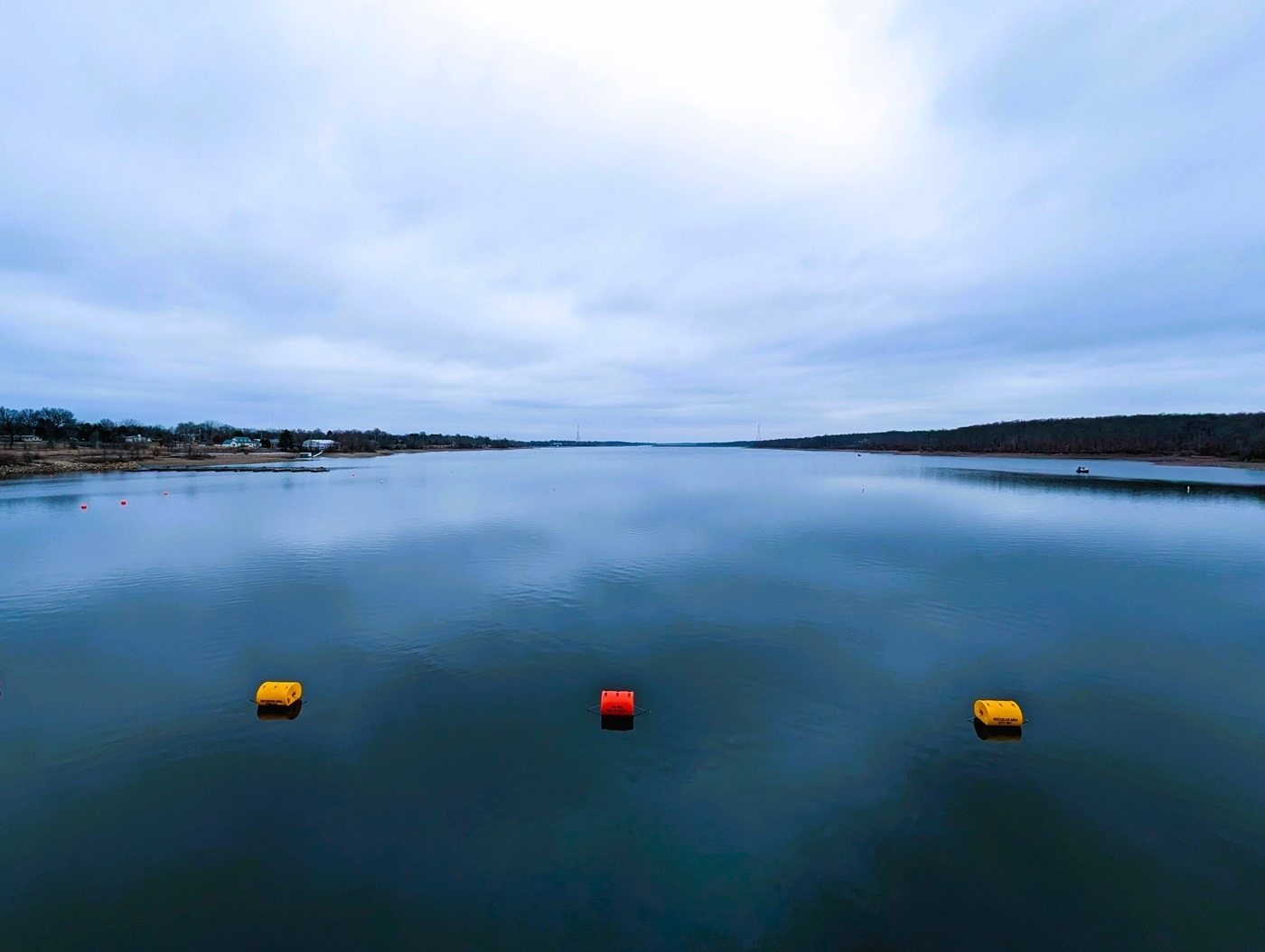
Comments are closed.